Before diving into Lightning Web Components (LWC), it’s crucial to understand the modern JavaScript features provided by ECMAScript standards. These features make your code more efficient, readable, and maintainable. This beginner’s guide will introduce you to the 8 essential ECMAScript features that are fundamental when working with LWCs.
Introduction
Lightning Web Components is a modern framework for building user interfaces on the Salesforce platform. It leverages the latest ECMAScript standards to provide a more efficient and streamlined development experience. Understanding these JavaScript features is essential for anyone looking to develop with LWCs.
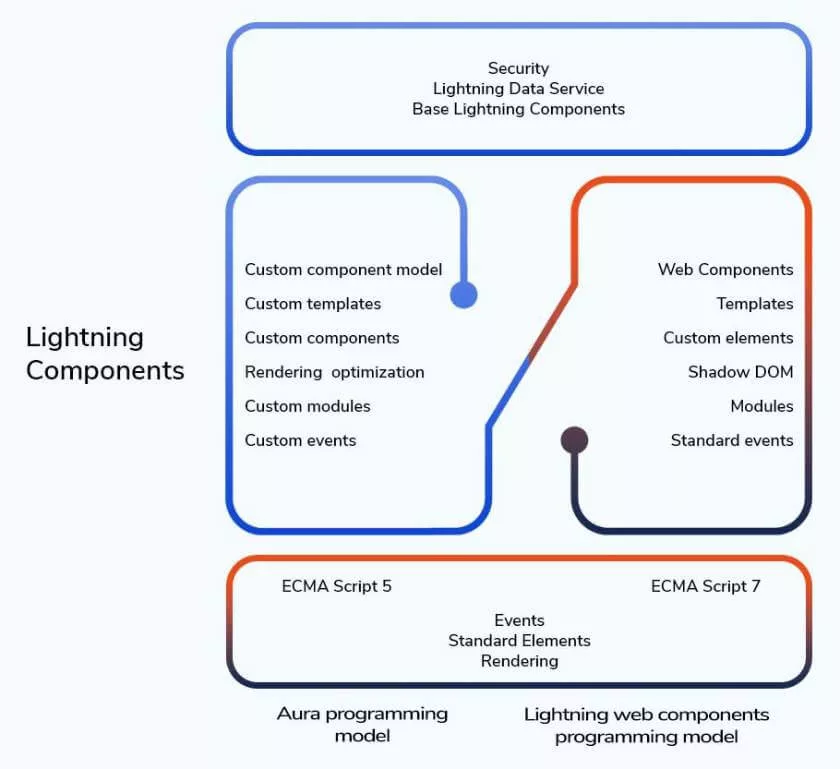
2. Why ECMAScript is Important for Lightning Web Components
ECMAScript is the standard that defines JavaScript. Over the years, new versions have introduced features that make the language more powerful and easier to use. Lightning Web Components are built using these modern JavaScript features, which means that familiarity with ECMAScript is vital for effective LWC development.
Benefits:
- Modern Syntax: Cleaner and more concise code.
- Enhanced Functionality: New features like modules and classes.
- Better Performance: Optimized code execution.
- Maintainability: Easier to read and maintain codebases.
Modules (import/export)
What Are Modules?
Modules allow you to break up your code into separate files, making it more organized and maintainable. In ECMAScript 6 (ES6), modules are implemented using the import
and export
statements.
How They Relate to LWC
In LWCs, each component is essentially a module. You use import
to bring in LightningElement from the lwc
module and other resources.
Example
myComponent.js
// Importing LightningElement from the lwc module
import { LightningElement } from 'lwc';
// Importing a utility function from another module
import { calculateTotal } from 'c/utils';
export default class MyComponent extends LightningElement {
total;
connectedCallback() {
this.total = calculateTotal(5, 10);
}
}
utils.js
// Exporting the utility function
export function calculateTotal(a, b) {
return a + b;
}
Key Points
- Use
export
to make functions, objects, or primitives available for import. - Use
import
to bring in code from other modules. - Helps in code reusability and organization.
Classes
What Are Classes?
Classes in JavaScript are syntactical sugar over the existing prototype-based inheritance model. They provide a clearer and more concise syntax for creating objects and dealing with inheritance.
How They Relate to LWC
Every LWC component is defined as a JavaScript class that extends LightningElement
.
Example
myComponent.js
import { LightningElement, api } from 'lwc';
export default class MyComponent extends LightningElement {
@api recordId;
get upperCaseRecordId() {
return this.recordId.toUpperCase();
}
}
Key Points
- Use the
class
keyword to define a class. - Use
extends
to inherit from another class. - Use the
@api
decorator to expose public properties and methods.
Arrow Functions
What Are Arrow Functions?
Arrow functions provide a shorter syntax for writing function expressions. They also lexically bind the this
value, which means they do not have their own this
.
How They Relate to LWC
Arrow functions are often used in LWCs for event handlers and iterators because they preserve the lexical scope.
Example
Using Arrow Functions
handleClick = () => {
// 'this' refers to the class instance
console.log('Button clicked:', this.recordId);
};
Using in Templates
<template>
<button onclick={handleClick}>Click Me</button>
</template>
Key Points
- Concise syntax:
() => { /* code */ }
. - Lexically binds
this
, which is useful in callbacks. - Cannot be used as constructors.
Template Literals
What Are Template Literals?
Template literals are string literals that allow embedded expressions. They are enclosed by backticks (`
) instead of quotes.
How They Relate to LWC
Template literals make it easier to create strings that include dynamic values, which is common in LWC components.
Example
const firstName = 'John';
const lastName = 'Doe';
// Using template literals
const fullName = `${firstName} ${lastName}`;
console.log(fullName); // Output: John Doe
Key Points
- Enclose strings with backticks:
`Your string here`
. - Embed expressions using
${expression}
. - Support multi-line strings without special characters.
Destructuring Assignment
What Is Destructuring?
Destructuring allows you to extract values from arrays or properties from objects into distinct variables.
How They Relate to LWC
Destructuring is useful when dealing with objects like event parameters or data from APIs in LWCs.
Example
Object Destructuring
const user = {
firstName: 'Jane',
lastName: 'Doe',
age: 28
};
const { firstName, age } = user;
console.log(firstName); // Output: Jane
console.log(age); // Output: 28
Array Destructuring
const numbers = [1, 2, 3];
const [one, two] = numbers;
console.log(one); // Output: 1
console.log(two); // Output: 2
Key Points
- Simplifies extraction of multiple properties.
- Can set default values.
- Works with nested objects and arrays.
Spread and Rest Operators
What Are Spread and Rest Operators?
The spread operator (...
) allows an iterable to expand in places where 0+ arguments are expected. The rest operator collects all remaining elements into an array.
How They Relate to LWC
Used in LWCs for copying objects, combining arrays, and handling variable numbers of arguments.
Example
Spread Operator
const arr1 = [1, 2, 3];
const arr2 = [4, 5];
const combinedArray = [...arr1, ...arr2];
console.log(combinedArray); // Output: [1, 2, 3, 4, 5]
Rest Operator
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3)); // Output: 6
Key Points
- Spread operator expands iterables into individual elements.
- Rest operator collects multiple elements into an array.
- Useful for cloning objects:
const clone = { ...original };
Promises and Async/Await
What Are Promises?
Promises represent the eventual completion (or failure) of an asynchronous operation and its resulting value.
What Is Async/Await?
Async/Await is syntactic sugar built on top of Promises, making asynchronous code look and behave more like synchronous code.
How They Relate to LWC
LWCs often interact with asynchronous operations, such as fetching data from Apex controllers or external APIs.
Example
Using Promises
// Calling an Apex method
import getContacts from '@salesforce/apex/ContactController.getContacts';
getContacts()
.then((result) => {
this.contacts = result;
})
.catch((error) => {
console.error(error);
});
Using Async/Await
async connectedCallback() {
try {
const result = await getContacts();
this.contacts = result;
} catch (error) {
console.error(error);
}
}
Key Points
- Promises have three states: pending, fulfilled, rejected.
async
functions return a Promise.- Use
await
to wait for a Promise to resolve.
Let and Const Declarations
What Are Let and Const?
let
and const
are block-scoped variable declaration keywords introduced in ES6, providing better scoping rules than var
.
How They Relate to LWC
Using let
and const
helps prevent common bugs related to variable hoisting and scope, making LWC code more reliable.
Example
Using let
for (let i = 0; i < 5; i++) {
// 'i' is only accessible within this block
console.log(i);
}
Using const
const MAX_LIMIT = 100;
// MAX_LIMIT = 200; // This will throw an error
Key Points
let
allows you to declare variables that are limited in scope to the block, statement, or expression.const
declares variables that are read-only after assignment.- Helps prevent accidental global variable declarations.
Conclusion
Understanding these 8 essential ECMAScript features is a significant step toward becoming proficient in Lightning Web Components development. These modern JavaScript capabilities not only make your code cleaner and more efficient but also align with best practices in LWC development.
Recap of Features
- Modules (import/export): Organize code into reusable pieces.
- Classes: Define component logic in a structured way.
- Arrow Functions: Write concise functions with lexical
this
. - Template Literals: Create strings with embedded expressions.
- Destructuring Assignment: Extract data from objects and arrays.
- Spread and Rest Operators: Expand and collect elements.
- Promises and Async/Await: Handle asynchronous operations elegantly.
- Let and Const Declarations: Use proper scoping for variables.
Next Steps
- Practice: Implement these features in small projects.
- Explore LWCs: Start building simple Lightning Web Components.
- Read Documentation: Familiarize yourself with the Salesforce LWC Developer Guide.
Additional Resources
- ECMAScript 6 Features: ECMAScript 6 — New Features: Overview & Comparison
- MDN Web Docs: JavaScript Reference
- Salesforce Trailhead: Lightning Web Components Basics
- YouTube Tutorials: Search for “Lightning Web Components tutorial” for visual learning.