Data abstraction is a programming concept which hides the internal details of system or object and shows through an interface. This approach is fully encapsulated and allows developers to interact with the system at a much higher level without needing (or wanting) to know all of these details. You have the benefit of better code maintenance and easier modification as it makes your programming easyInstead, because you care about what an object does rather than how objects work.
Data abstraction
Data abstraction divides the interface from its implementation that provides better modularity, reusability of code and security. It is a key concept in object-oriented programming (OOP) that helps to create more reusable, maintainable and scalable systems.
Data abstraction is a mechanism of retrieving the essentials details without dealing with background details.
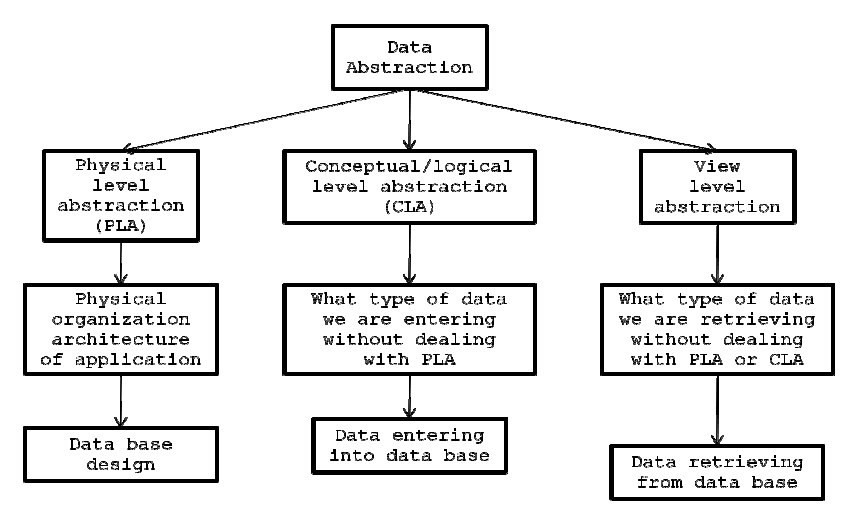
In real world we have three levels of abstractions. They are physical level abstractions. They are physical level abstraction, conceptual/level abstraction and view level abstraction.
- Physical level abstraction is one, it always deals with physical organization architecture of the application. For example in real world an application designing of any problem comes under physical level abstraction.
- Conceptual/logical level abstraction is one it always deals with kind of data we are entering without dealing with physical architecture of the application. For example entering the data into the database, writing the coding and applying testing principle comes under conceptual level abstraction.
- View level abstraction deals with what kind of data we are retrieving without dealing with both conceptual level abstraction and physical level abstraction. For example retrieving the data from the database in various combinations. All internet users come under view level abstraction.
Examples for Data abstraction
Data Abstraction is a fundamental concept in object-oriented programming (OOP) where only essential details of an object are shown to the user, while unnecessary details are hidden. This simplifies the user’s interaction with the data, making it easier to work with complex systems by focusing only on what is necessary.
Simple Example for Beginners
Consider a Coffee Machine. When you press a button to make coffee, you don’t need to know the detailed inner workings of the machine, like how it heats water or mixes coffee powder. All you need to know are the simple instructions for making coffee. The complex processes are hidden, providing only a simple interface for interaction.
Code Example in Python
Here’s a beginner-friendly example of data abstraction using a Bank Account class:
class BankAccount:
def __init__(self, account_holder, balance=0):
self.account_holder = account_holder # Public attribute
self.__balance = balance # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
print(f"${amount} deposited successfully.")
else:
print("Deposit amount must be positive.")
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
print(f"${amount} withdrawn successfully.")
else:
print("Insufficient balance or invalid amount.")
def get_balance(self):
return f"Current balance: ${self.__balance}" # Returns balance without exposing internal details
# Using the BankAccount class
my_account = BankAccount("John Doe", 100)
my_account.deposit(50)
print(my_account.get_balance())
my_account.withdraw(30)
print(my_account.get_balance())
Explanation
- Private Attribute (
__balance
): The__balance
attribute is private, meaning it cannot be accessed directly outside the class (e.g.,my_account.__balance
will not work). This hides unnecessary details from the user, like the exact balance data. - Public Methods (
deposit
,withdraw
,get_balance
): These methods provide a way to interact with theBankAccount
class, abstracting away complex internal processes. Users can deposit, withdraw, or check the balance without needing to know how balance calculations work. - Abstraction in Action: The class provides only essential methods, hiding details (like directly modifying balance) and exposing only what’s needed for simple interaction.
This is data abstraction: the user interacts with a simplified, user-friendly interface while complex details are hidden within the class structure.