Salesforce is a robust Customer Relationship Management (CRM) platform widely used by businesses to manage customer data, sales processes, and marketing campaigns. Developers and administrators often encounter challenges while customizing and optimizing Salesforce to meet specific business needs. This guide compiles some of Top Salesforce Interview Questions and their answers, providing valuable insights and solutions to common Salesforce issues.
Top Salesforce Interview Questions
Prepare for your next job with our Top Salesforce Interview Questions, featuring expert insights and commonly asked questions from leading MNCs.
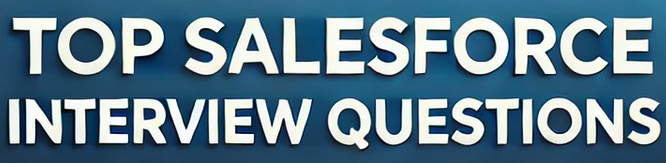
Understanding Governor Limits in Apex
Question:
What are Governor Limits in Salesforce, and how can I avoid hitting them in my Apex code?
Answer:
Governor Limits are runtime limits enforced by the Salesforce platform to ensure efficient resource usage and to prevent any single tenant from monopolizing shared resources. These limits are crucial in a multi-tenant environment like Salesforce.
Common Governor Limits Include:
- SOQL Queries: Maximum of 100 SOQL queries per synchronous transaction.
- DML Statements: Maximum of 150 DML statements per transaction.
- Heap Size: Maximum of 6 MB for synchronous and 12 MB for asynchronous transactions.
- CPU Time: Maximum CPU time on the Salesforce servers is 10,000 milliseconds for synchronous transactions.
Strategies to Avoid Governor Limits:
- Bulkify Your Code: Write code that can handle multiple records at once. Use collections like Lists or Maps to process records in bulk.
// Example of bulkified trigger
trigger AccountTrigger on Account (before insert, before update) {
for (Account acc : Trigger.new) {
// Process each account
}
}
- Use Efficient SOQL Queries: Avoid placing SOQL queries inside loops. Retrieve all necessary data in a single query before the loop.
// Bad practice
for (Account acc : accountList) {
List<Contact> contacts = [SELECT Id FROM Contact WHERE AccountId = :acc.Id];
}
// Good practice
Set<Id> accountIds = new Set<Id>();
for (Account acc : accountList) {
accountIds.add(acc.Id);
}
List<Contact> contacts = [SELECT Id, AccountId FROM Contact WHERE AccountId IN :accountIds];
- Optimize Loops and Collections: Use Maps and Sets to store and retrieve data efficiently.
- Implement Pagination: When dealing with large datasets in Visualforce pages or Lightning components, implement pagination to limit the number of records processed at a time.
- Leverage Asynchronous Processing: Use Batch Apex, Queueable Apex, or Future methods to process large volumes of data asynchronously.
- Use @ReadOnly Annotation: For read-only operations that don’t require DML, use the @ReadOnly annotation to increase the limit of records retrieved.
Best Practices for Writing Triggers
Question:
What are the best practices for writing Apex triggers in Salesforce?
Answer:
Apex triggers are essential for performing custom actions before or after events on records. Following best practices ensures that triggers are efficient, maintainable, and scalable.
Best Practices:
- One Trigger Per Object: Create a single trigger for each object to manage all related events. This approach simplifies maintenance and avoids unintended behavior from multiple triggers.
- Use Trigger Handler Classes: Delegate logic from triggers to handler classes. This separation of concerns improves readability and allows for better code organization.
trigger AccountTrigger on Account (before insert, before update) {
AccountTriggerHandler.handleTrigger(Trigger.new, Trigger.oldMap);
}
- Bulkify Code: Ensure that the trigger can handle bulk operations, processing multiple records efficiently.
- Avoid Hardcoding IDs and Values: Use Custom Settings or Custom Metadata Types to store configuration data instead of hardcoding values.
- Handle All Trigger Contexts: Account for all trigger contexts (before insert, after insert, before update, etc.) even if they are not currently used.
- Prevent Recursion: Implement logic to prevent recursive trigger execution, which can lead to hitting governor limits or unexpected behavior.
- Write Test Classes: Ensure that triggers have comprehensive test coverage, including positive and negative test cases.
Working with SOQL and SOSL Queries
Question:
How can I optimize SOQL queries to improve performance and avoid governor limits?
Answer:
Optimizing SOQL queries is crucial for performance and resource management.
Optimization Techniques:
- Selective Queries: Use selective filters in WHERE clauses to reduce the number of records returned.
// Use indexed fields in WHERE clause
List<Account> accounts = [SELECT Id, Name FROM Account WHERE Industry = 'Technology'];
- Avoid Wildcard SELECT: Specify only the fields you need instead of using SELECT *.
- Use LIMIT Clause: Restrict the number of records returned when appropriate.
List<Contact> contacts = [SELECT Id, Name FROM Contact WHERE AccountId = :accountId LIMIT 100];
- Leverage Relationship Queries: Use parent-child relationships to retrieve related records efficiently.
// Query contacts related to accounts
List<Account> accounts = [SELECT Id, Name, (SELECT Id, Name FROM Contacts) FROM Account WHERE Id IN :accountIds];
- Use SOSL for Text Searches: When searching for text across multiple objects and fields, use SOSL instead of multiple SOQL queries.
List<List<sObject>> searchResults = [FIND 'Acme' IN ALL FIELDS RETURNING Account(Id, Name), Contact(Id, Name)];
- Index Custom Fields: If you frequently filter on a custom field, consider indexing it to improve query performance.
- Monitor Query Plans: Use the Query Plan Tool in the Developer Console to analyze and optimize queries.
Implementing Batch Apex for Large Data Volumes
Question:
How do I use Batch Apex to process large volumes of data without hitting governor limits?
Answer:
Batch Apex allows you to process records asynchronously in manageable chunks, which is ideal for operations that exceed governor limits.
Implementing Batch Apex:
- Create a Batch Class:
global class AccountBatchProcessor implements Database.Batchable<sObject> {
global Database.QueryLocator start(Database.BatchableContext BC) {
return Database.getQueryLocator('SELECT Id, Name FROM Account');
}
global void execute(Database.BatchableContext BC, List<Account> scope) {
// Process each batch of accounts
for (Account acc : scope) {
// Perform operations
}
update scope;
}
global void finish(Database.BatchableContext BC) {
// Post-processing if needed
}
}
- Execute the Batch Class:
// Execute batch with a batch size of 200
AccountBatchProcessor batch = new AccountBatchProcessor();
Database.executeBatch(batch, 200);
Key Considerations:
- Batch Size: Default batch size is 200 records. Adjust based on processing complexity to optimize performance.
- Governor Limits Reset: Each batch execution is a discrete transaction, so governor limits are reset between batches.
- Chaining Batches: Use the
finish
method to chain batch executions if necessary. - Test Methods: Write test methods to cover batch classes, ensuring at least 75% code coverage.
Creating and Using Custom Metadata Types
Question:
What are Custom Metadata Types in Salesforce, and how can I use them to store configuration data?
Answer:
Custom Metadata Types allow you to define application metadata that can be packaged and deployed across environments. They are similar to Custom Settings but offer additional benefits, such as being included in managed packages and supporting metadata relationships.
Creating Custom Metadata Types:
- Define the Metadata Type:
- Navigate to Setup > Custom Metadata Types.
- Click New Custom Metadata Type.
- Specify the Label, Plural Label, and Object Name.
- Add Custom Fields:
- Define custom fields to store configuration data, similar to how you add fields to custom objects.
- Create Metadata Records:
- Click Manage Records next to your Custom Metadata Type.
- Create records with the necessary configuration values.
Using Custom Metadata in Apex:
- Query Custom Metadata using SOQL.
List<My_Metadata_Type__mdt> configs = [SELECT DeveloperName, Field_One__c, Field_Two__c FROM My_Metadata_Type__mdt];
- Access metadata in code to control application behavior.
Advantages over Custom Settings:
- Deployable: Custom Metadata Types and their records can be deployed via change sets or packages.
- Protected Metadata: You can mark fields as protected to prevent subscriber access.
- Relationships: Support for metadata relationships allows for referencing other metadata.
Building Lightning Web Components (LWC)
Question:
How do I create a Lightning Web Component, and what are the benefits over Aura Components?
Answer:
Lightning Web Components (LWC) are the modern standard for building components in Salesforce, leveraging native web standards for better performance.
Creating an LWC:
- Set Up Development Environment:
- Install Salesforce CLI.
- Use Visual Studio Code with the Salesforce Extension Pack.
- Create an LWC Project:
sfdx force:project:create -n myLWCProject
- Generate a New Component:
sfdx force:lightning:component:create -n myComponent -d force-app/main/default/lwc
- Component Structure:
- HTML File: Template for the component’s UI.
- JavaScript File: Component’s logic.
- XML Configuration File: Metadata for the component.
- Deploy to Org:
sfdx force:source:push
Benefits of LWC over Aura Components:
- Performance: Faster load times due to minimal abstraction and utilization of native browser APIs.
- Standardized: Aligns with modern web development practices.
- Reusable Components: Easier to create and manage reusable components.
- Improved Developer Experience: Simplified syntax and better tooling support.
Integrating Salesforce with External Systems
Question:
What are the methods for integrating Salesforce with external systems, and how do I choose the right one?
Answer:
Salesforce provides multiple integration methods to connect with external systems.
Integration Methods:
- REST API:
- Use for lightweight, stateless operations.
- Supports JSON and XML formats.
- Ideal for web and mobile applications.
- SOAP API:
- Suitable for enterprise-level integrations requiring formal contracts.
- Uses XML.
- Supports complex transactions and stateful operations.
- Outbound Messaging:
- Configured in Workflow Rules or Processes.
- Sends SOAP messages to external services when records meet criteria.
- Apex Callouts:
- Use Apex to make HTTP requests to external services.
- Supports RESTful services and web services.
HttpRequest request = new HttpRequest();
request.setEndpoint('https://api.example.com/data');
request.setMethod('GET');
Http http = new Http();
HttpResponse response = http.send(request);
- Platform Events:
- Publish/subscribe model for event-driven architectures.
- Asynchronous communication between Salesforce and external systems.
- Salesforce Connect:
- Access external data in real-time using External Objects.
- Supports OData 2.0 and 4.0 protocols.
Choosing the Right Method:
- Data Volume and Frequency: For real-time data, use APIs or Platform Events. For periodic updates, consider batch processes.
- Direction of Data Flow: Determine if data is inbound to Salesforce, outbound, or bidirectional.
- Complexity and Requirements: For simple integrations, REST API may suffice. For complex transactions, SOAP API or Salesforce Connect might be better.
- External System Compatibility: Ensure the external system supports the chosen integration method.
Handling Asynchronous Processes with Future Methods and Queues
Question:
When should I use Future methods, Queueable Apex, or Batch Apex for asynchronous processing?
Answer:
Asynchronous processing allows long-running operations to be performed without blocking the user interface.
Future Methods:
- Use Cases:
- Callouts to external services.
- Operations that need to run in the background.
- Limitations:
- Cannot chain future methods.
- Limited to primitive data types as parameters.
@future
public static void performAsyncOperation() {
// Asynchronous logic
}
Queueable Apex:
- Advantages:
- Supports non-primitive types as parameters.
- Allows job chaining.
- Use Cases:
- When you need more complex asynchronous processing than Future methods allow.
public class AsyncJob implements Queueable {
public void execute(QueueableContext context) {
// Asynchronous logic
}
}
// Enqueue the job
System.enqueueJob(new AsyncJob());
Batch Apex:
- Use Cases:
- Processing large volumes of records.
- Operations that exceed governor limits in synchronous context.
- Features:
- Can be scheduled.
- Supports Stateful interfaces for maintaining state between batches.
Choosing Between Them:
- Simple Asynchronous Task: Use Future methods.
- Need Chaining or Complex Data Types: Use Queueable Apex.
- Large Data Volume Processing: Use Batch Apex.
Utilizing Salesforce Flow for Automation
Question:
How can I use Salesforce Flow to automate business processes without code?
Answer:
Salesforce Flow is a powerful tool that allows administrators to automate complex business processes using a visual interface.
Types of Flows:
- Screen Flows: Guide users through a series of screens to collect and update data.
- Autolaunched Flows: Run in the background when triggered by a process or Apex.
Creating a Flow:
- Define the Flow Type:
- Choose between Screen Flow or Autolaunched Flow based on requirements.
- Add Elements:
- Interaction Elements: Screen, Action, Subflow.
- Logic Elements: Assignment, Decision, Loop.
- Data Elements: Create Records, Update Records, Get Records, Delete Records.
- Configure Elements:
- Set variables, formulas, and define logic paths.
- Activate the Flow:
- Once tested, activate the flow for use.
Triggering Flows:
- Button or Link: Users initiate the flow.
- Record Changes: Triggered when records are created or updated.
- Platform Events: Respond to events published in Salesforce.
Benefits:
- No Code Required: Ideal for administrators without coding experience.
- Complex Logic: Can handle complex branching and data manipulation.
- Maintainability: Easier to update compared to Apex code.
Best Practices for Data Migration and Management
Question:
What are the best practices for migrating data into Salesforce and managing it effectively?
Answer:
Data migration involves transferring data from external systems into Salesforce. Effective management ensures data integrity and accessibility.
Best Practices:
- Data Mapping:
- Map fields from the source system to Salesforce objects and fields.
- Account for required fields and data types.
- Data Cleansing:
- Clean data before importing to remove duplicates and correct errors.
- Standardize data formats (e.g., dates, phone numbers).
- Use Data Loader or Data Import Wizard:
- Data Loader: For large volumes of data and complex mappings.
- Data Import Wizard: For simple imports up to 50,000 records.
- Handle Relationships:
- Import parent records first (e.g., Accounts), then child records (e.g., Contacts).
- Use external IDs or Salesforce IDs to establish relationships.
- Validation Rules and Triggers:
- Disable or adjust validation rules and triggers during migration to prevent errors.
- Re-enable after data import and run checks.
- Backup Data:
- Always back up existing Salesforce data before importing new data.
- Test in Sandbox:
- Perform a test migration in a sandbox environment to identify issues.
- Data Governance:
- Establish policies for data quality, ownership, and access.
- Regularly audit and cleanse data.
Implementing Security and Sharing Models
Question:
How do I set up security and sharing settings in Salesforce to control user access to data?
Answer:
Salesforce provides a layered security model to control access at various levels.
Security Layers:
- Organization-Level Security:
- Trusted IP Ranges: Define IP ranges from which users can access Salesforce.
- Login Hours: Set login hours for profiles.
- Object-Level Security:
- Profiles and Permission Sets: Control access (Create, Read, Edit, Delete) to objects.
- Field-Level Security:
- Field Permissions: Restrict access to specific fields on an object.
- Record-Level Security:
- Organization-Wide Defaults (OWD): Set the default sharing settings for objects (Private, Public Read Only, Public Read/Write).
- Role Hierarchy: Users inherit access to records owned by or shared with users below them.
- Sharing Rules: Extend access to users based on criteria or ownership.
- Manual Sharing: Users can share individual records with others.
Setting Up Security:
- Define Roles and Profiles:
- Create profiles to define object and field permissions.
- Assign users to roles for record-level access.
- Configure OWD:
- Determine the baseline level of access for each object.
- Set Up Sharing Rules:
- Create rules to share records with roles, groups, or users based on criteria.
- Use Permission Sets:
- Grant additional permissions to specific users without changing their profiles.
Best Practices:
- Principle of Least Privilege: Give users the minimum access necessary to perform their jobs.
- Regular Audits: Review security settings periodically to ensure compliance.
- Training: Educate users about data security and their responsibilities.
Testing and Debugging in Salesforce
Question:
How can I effectively test and debug my Apex code and Salesforce configurations?
Answer:
Testing and debugging are crucial for ensuring code quality and system reliability.
Testing Apex Code:
- Write Test Classes:
- All Apex code must have test coverage of at least 75% before deployment.
- Test classes should cover various scenarios, including positive, negative, and bulk operations.
- Use Test.startTest() and Test.stopTest():
- Isolate the code being tested and reset governor limits within test methods.
@isTest
public class MyClassTest {
@isTest
static void testMyMethod() {
Test.startTest();
// Call methods to test
Test.stopTest();
// Assert results
}
}
- Use Mocking for Callouts:
- Use HttpCalloutMock or WebServiceMock interfaces to simulate callouts in tests.
Debugging Techniques:
- Debug Logs:
- Set up debug logs to capture system operations, errors, and variable values.
- Use System.debug() statements to output variable values.
- Developer Console:
- Use the Developer Console to execute anonymous Apex, inspect variables, and analyze logs.
- Checkpoint Inspector:
- Set checkpoints in the Developer Console to inspect the state of variables at specific points.
- Apex Replay Debugger:
- Use in Visual Studio Code to replay debug logs and inspect execution flow.
Testing Configurations:
- Validation Rules and Workflow:
- Test to ensure that validation rules and workflow rules behave as expected.
- Process Builder and Flows:
- Test processes and flows with different data inputs.
- Security Settings:
- Verify user access by testing with different user profiles and permissions.
Optimizing Performance in Salesforce Applications
Question:
What strategies can I use to optimize the performance of my Salesforce applications?
Answer:
Optimizing performance enhances user experience and system efficiency.
Strategies:
- Efficient Queries:
- Optimize SOQL queries by using selective filters and indexes.
- Avoid unnecessary queries and data retrieval.
- Bulkify Code:
- Ensure code can handle large data volumes without hitting governor limits.
- Asynchronous Processing:
- Offload heavy processing to asynchronous methods when possible.
- Caching Data:
- Use platform cache to store frequently accessed data.
- Optimize Visualforce Pages and Lightning Components:
- Minimize view state size in Visualforce pages.
- Use Lightning Data Service in Lightning components to reduce server calls.
- Static Resources:
- Serve images, scripts, and stylesheets from static resources to improve load times.
- Reduce Client-Side Processing:
- Optimize JavaScript code and minimize DOM manipulation.
- Limitations Awareness:
- Be aware of limits like CPU time and heap size, and optimize code accordingly.
Conclusion
For professionals preparing to advance their careers, we’re excited to announce our upcoming compilation of Top Salesforce Interview Questions. This resource will feature the most recent and frequently asked questions from top multinational companies (MNCs), providing you with valuable insights and answers to help you excel in interviews. Stay tuned for updates as we gather the latest industry trends and topics to keep you ahead in your Salesforce journey.