Apex Operators list
In our last Salesforce Developer Tutorial we have learned about Apex operators declaration and Apex data types. In this Salesforce Tutorial we are going to learn about Apex operators and their list.
Apex Operators and Apex Operators List.
Apex language supports some list of standard operators that are support by many other programming languages other than Apex language. When learning about Apex operators we have to get some knowledge on operands and their precedence. When any two operators are used in an expression then the operator which has lower precedence is evaluated first and then the second operator is evaluated.
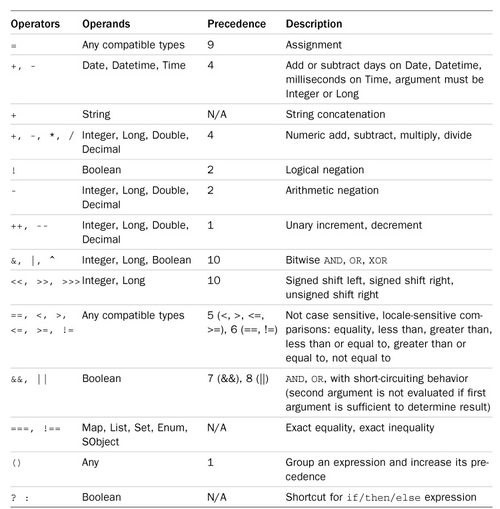
The above are the list of operators, operands, precedence values and their description.
Here’s a table outlining the most common Apex operators used in Salesforce, along with explanations in natural language to help beginners understand how each operator functions.
Apex Operators List
Operator Type | Operator | Description | Example |
---|---|---|---|
Arithmetic | + | Adds two values. Used for numeric addition or string concatenation. | a + b or "Hello " + "World" |
- | Subtracts the second value from the first. | a - b | |
* | Multiplies two values. | a * b | |
/ | Divides the first value by the second. | a / b | |
% | Modulus operator returns the remainder of a division operation. | a % b | |
Assignment | = | Assigns a value to a variable. | x = 10 |
+= | Adds the right value to the left variable and assigns the result to the left variable. | x += 5 (same as x = x + 5 ) | |
-= | Subtracts the right value from the left variable and assigns the result to the left variable. | x -= 3 (same as x = x - 3 ) | |
*= | Multiplies the left variable by the right value and assigns the result to the left variable. | x *= 2 (same as x = x * 2 ) | |
/= | Divides the left variable by the right value and assigns the result to the left variable. | x /= 4 (same as x = x / 4 ) | |
Comparison | == | Checks if two values are equal. Returns true if they are, false otherwise. | a == b |
!= | Checks if two values are not equal. | a != b | |
> | Checks if the left value is greater than the right. | a > b | |
< | Checks if the left value is less than the right. | a < b | |
>= | Checks if the left value is greater than or equal to the right. | a >= b | |
<= | Checks if the left value is less than or equal to the right. | a <= b | |
Logical | && | Logical AND. Returns true if both conditions are true. | a > 0 && b > 0 |
|| | Logical OR. Returns true if at least one of the conditions is true. | a > 0 || b > 0 | |
! | Logical NOT. Reverses the boolean value (true becomes false, false becomes true). | !isAvailable | |
Ternary | ?: | A shorthand for if-else . If a condition is true, it returns the first value; otherwise, it returns the second. | x = (a > b) ? a : b |
Increment/Decrement | ++ | Increments a variable’s value by 1. Can be used in both prefix (++x ) and postfix (x++ ) forms. | x++ or ++x |
-- | Decrements a variable’s value by 1. Can be used in both prefix (--x ) and postfix (x-- ) forms. | x-- or --x | |
Bitwise | & | Bitwise AND. Compares each bit of two integers. | a & b |
| | Bitwise OR. Compares each bit of two integers and returns 1 if either bit is 1. | a | b | |
^ | Bitwise XOR. Compares each bit and returns 1 if the bits are different. | a ^ b | |
~ | Bitwise NOT. Inverts each bit (0 becomes 1, 1 becomes 0). | ~a | |
<< | Left Shift. Shifts the bits to the left by the specified number of positions. | a << 2 (shifts a two bits left) | |
>> | Right Shift. Shifts the bits to the right by the specified number of positions. | a >> 2 (shifts a two bits right) | |
Instanceof | instanceof | Checks if an object is an instance of a specific class or interface. Returns true if it is. | if (x instanceof Account) |
Explanation of Common Apex Operators
- Arithmetic Operators: Used for mathematical calculations. For example,
+
can add numbers or concatenate strings.%
is particularly useful for checking if a number is even or odd (e.g.,num % 2 == 0
for even numbers). - Assignment Operators: These operators assign values to variables. Combined assignment operators like
+=
and-=
make it easy to modify a variable’s value without rewriting the variable on both sides of the expression. - Comparison Operators: These operators are used in conditional statements to compare values. For instance,
a == b
checks ifa
is equal tob
. These are commonly used inif
statements to control program flow. - Logical Operators: Often used in conditional expressions, these operators combine boolean conditions. For example,
a > 0 && b > 0
checks if botha
andb
are positive. Logical operators are essential in constructing complex conditions. - Ternary Operator: The
?:
operator is a compact form ofif-else
and is useful for setting values conditionally in a single line. For example,max = (a > b) ? a : b
setsmax
to the larger ofa
orb
. - Increment/Decrement Operators: These operators are shortcuts for adding or subtracting 1 from a variable.
x++
increasesx
by 1, andx--
decreases it by 1. The prefix and postfix forms can have different effects in expressions. - Bitwise Operators: These operators perform operations on the binary representation of numbers. For instance,
a & b
performs an AND operation on each bit ofa
andb
. These are advanced operators typically used in low-level programming or specialized calculations. - Instanceof Operator: This operator checks if an object belongs to a specific class or implements an interface. It is useful for ensuring that a variable is of a particular type before performing operations on it.
By understanding and practicing these operators, you can effectively manipulate data, control logic, and implement more complex solutions within Salesforce Apex. Each operator has specific use cases and can simplify the code when used appropriately.